control 폴더를 새로 만들어 작업한다.
welcome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>환영합니다</title>
</head>
<body>
<strong>대한민국</strong>
<hr>
<span style='color:red'>오필승코리아</span>
<hr>
<table border='1'>
<tr>
<th>이름</th>
<td>무궁화</td>
</tr>
</table>
</body>
</html>
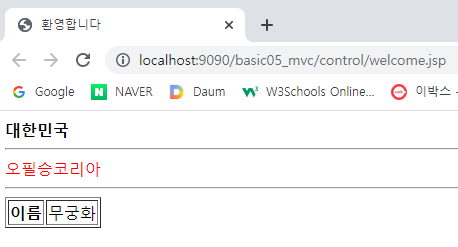

사용자가 페이지를 요청할 때 문서로 요청할 수도 있지만 아래와 같이 명령어로도 요청할 수 있다.
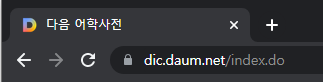
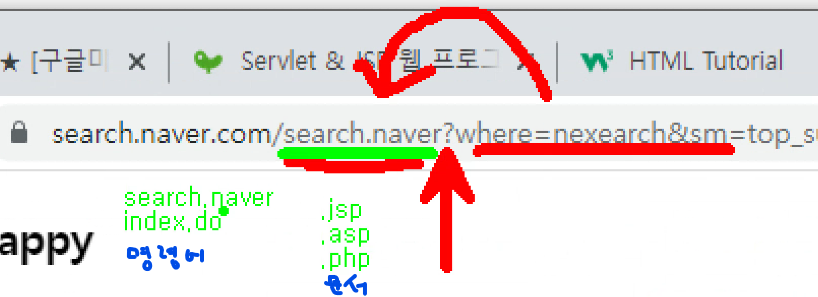
패키지와 클래스를 만든다.
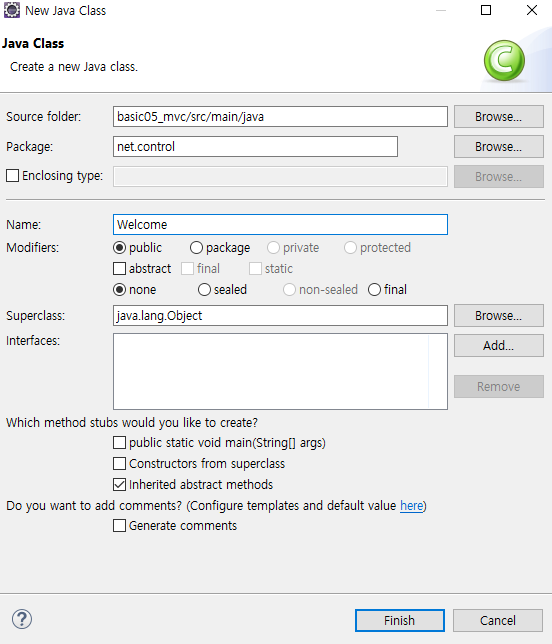
HttpServlet 클래스
https://docs.oracle.com/javaee/7/api/
Java(TM) EE 7 Specification APIs
docs.oracle.com
http 프로토콜 기반의 요청과 응답을 처리해주는 클래스
HttpServlet 클래스를 상속받아 Welcome 클래스를 만들 것이다. 결과 확인은 http://localhost:9090/basic05_mvc/wel.do 여기서 할 것이고 wel.do는 등록할 명령어로 원하는 대로 이름을 정하면 된다.
web.xml
<!-- Welcome 서블릿 등록 -->
<servlet>
<servlet-name></servlet-name>
<servlet-class>net.control.Welcome</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name></servlet-name>
<url-pattern>/wel.do</url-pattern>
</servlet-mapping>
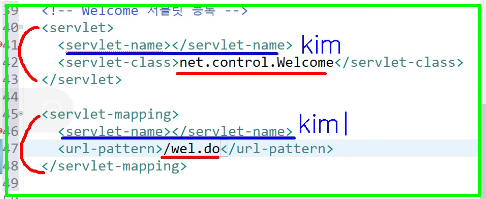
servlet-name은 두 개의 부분이 한 몸이라는 것을 연결해주기 위해 사용한다.
Welcome.java
package net.control;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/*
참조 https://docs.oracle.com/javaee/7/api/
● HttpServlet 클래스
- http 프로토콜 기반의 요청과 응답을 처리해주는 클래스
● 결과 확인
- http://localhost:9090/basic05_mvc/wel.do
● Welcome 서블릿 클래스
- web.xml (배치 관리자)에 등록해야 한다.
*/
public class Welcome extends HttpServlet{
//나의 클래스의 부모가 class : extends 상속(확장)
//나의 클래스의 부모가 interface : implements 상속(구현)
@Override //<- 재정의(리폼)
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//전송방식 method=get방식으로 요청하면 doGet() 함수가 자동으로 호출됨
//req : 모델1방식의 내부객체 request와 동일
//resp : 모델1방식의 내부객체 response와 동일
try {
//HTML 문서 구조로 응답
resp.setContentType("text/html; charset=UTF-8");
//단순 문자열 응답(AJAX)
//resp.setContentType("text/plain; charset=UTF-8");
//요청한 사용자에게 응답하기 위한 출력 객체
PrintWriter out = resp.getWriter();
out.print(" <!DOCTYPE html> ");
out.print(" <html> ");
out.print(" <head> ");
out.print(" <meta charset='UTF-8'> ");
out.print(" <title>환영합니다</title> ");
out.print(" </head> ");
out.print(" <body> ");
out.print(" <strong>대한민국</strong> ");
out.print(" <hr> ");
out.print(" <span style='color:red'>오필승코리아</span> ");
out.print(" <hr> ");
out.print(" <table border='1'> ");
out.print(" <tr> ");
out.print(" <th>이름</th> ");
out.print(" <td>무궁화</td> ");
out.print(" </tr> ");
out.print(" </table> ");
out.print(" </body> ");
out.print(" </html> ");
} catch (Exception e) {
System.out.println("요청실패 : " + e);
}//end
}//doGet() end
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//전송방식 method=post방식으로 요청하면 doPost() 함수가 자동으로 호출됨
}//doPost() end
}//class end
위에서 볼 수 있듯이 서블릿으로만 페이지를 만들면 HTML을 출력하는 것이 불편하다.
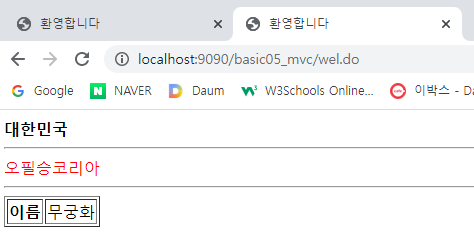
LifeCycle.java
package net.control;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/*
● HttpServlet 클래스의 계층도
class GenericServlet {}
class HttpServlet extends GenericServlet {}
class LifeCycle extends HttpServlet {}
● HttpServlet 생명주기-----------------------------
init() 서블릿이 호출되면 1번만 호출
-> service()
-> service()
-> service() 사용자가 요청할 때마다 계속 호출 (doGet()과 doPost()를 구분하는 역할)
-> service()
-> service()
destroy() 서버가 중지되면 1번만 호출
------------------------------------------------
● LifeCycle 서블릿을 /WEB-INF/web.xml에 등록해야 함
● 결과 확인 http://localhost:9090/basic05_mvc/life.do
*/
public class LifeCycle extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//method=get 방식으로 요청하면 service()함수가 호출함
super.doGet(req, resp);
System.out.println("doGet()호출...");
}//doGet() end
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//method=post 방식으로 요청하면 service()함수가 호출함
super.doPost(req, resp);
System.out.println("doPost()호출...");
}//doPost() end
@Override
protected void service(HttpServletRequest arg0, HttpServletResponse arg1) throws ServletException, IOException {
//URL을 통해서 요청이 들어오면, 전송방식이 method=get인지 method=post인지 판단해서
//get 방식이면 doGet(), post 방식이면 doPost()를 호출해 주는 함수
super.service(arg0, arg1);
System.out.println("service()호출...");
}//service() end
@Override
public void destroy() {
//서버가 중지되면 자동으로 1번만 호출
super.destroy();
System.out.println("destroy()호출...");
}//destroy() end
@Override
public void init() throws ServletException {
//서블릿이 최초로 호출될 때 1번만 호출
//초기 환경 설정할 때 사용
super.init();
System.out.println("init()호출...");
}//init() end
}//class end
web.xml
<!-- LifeCycle서블릿(HttpServlet 생명주기) 등록 -->
<servlet>
<servlet-name>lc</servlet-name>
<servlet-class>net.control.LifeCycle</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>lc</servlet-name>
<url-pattern>/life.do</url-pattern>
</servlet-mapping>
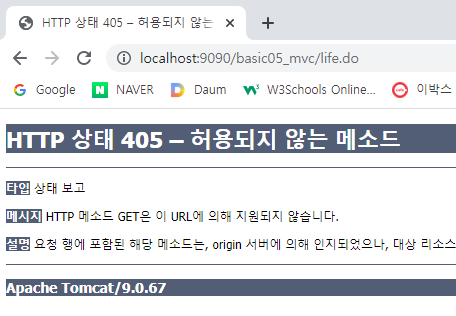
위 창을 새로고침 할 때마다
콘솔창에 doGet()호출... service()호출... 문구가 출력된다. 그리고 서버를 중지하면 destroy()호출... 문구가 출력된다.
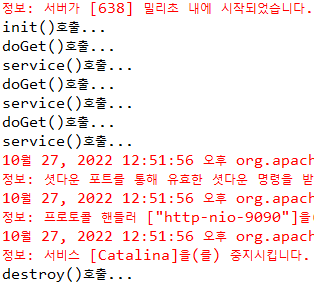
'웹개발 교육 > jsp' 카테고리의 다른 글
[65일] jsp (47) - MVC 패턴 (페이지 이동) (0) | 2022.10.28 |
---|---|
[64일] jsp (46) - MVC 패턴 (페이지 이동) (0) | 2022.10.27 |
[63일] jsp (44) - JSTL (0) | 2022.10.26 |
[63일] jsp (43) - EL (0) | 2022.10.26 |
[63일] jsp (42) - error (0) | 2022.10.26 |
control 폴더를 새로 만들어 작업한다.
welcome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>환영합니다</title>
</head>
<body>
<strong>대한민국</strong>
<hr>
<span style='color:red'>오필승코리아</span>
<hr>
<table border='1'>
<tr>
<th>이름</th>
<td>무궁화</td>
</tr>
</table>
</body>
</html>
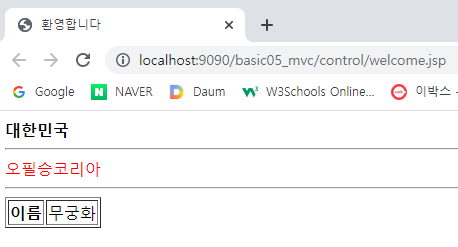

사용자가 페이지를 요청할 때 문서로 요청할 수도 있지만 아래와 같이 명령어로도 요청할 수 있다.
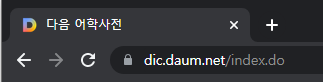
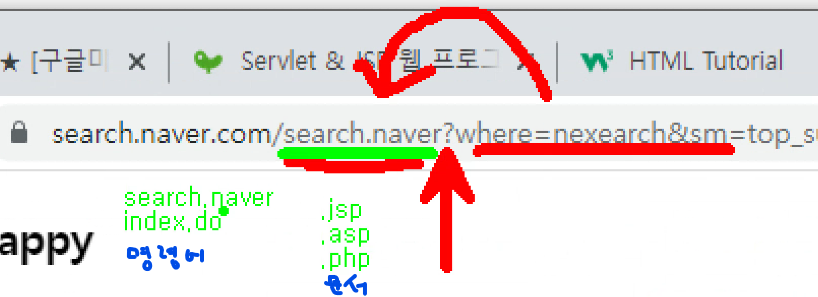
패키지와 클래스를 만든다.
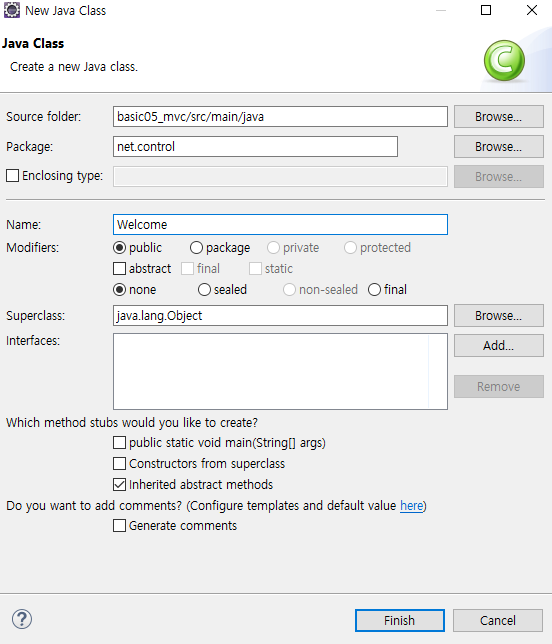
HttpServlet 클래스
https://docs.oracle.com/javaee/7/api/
Java(TM) EE 7 Specification APIs
docs.oracle.com
http 프로토콜 기반의 요청과 응답을 처리해주는 클래스
HttpServlet 클래스를 상속받아 Welcome 클래스를 만들 것이다. 결과 확인은 http://localhost:9090/basic05_mvc/wel.do 여기서 할 것이고 wel.do는 등록할 명령어로 원하는 대로 이름을 정하면 된다.
web.xml
<!-- Welcome 서블릿 등록 -->
<servlet>
<servlet-name></servlet-name>
<servlet-class>net.control.Welcome</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name></servlet-name>
<url-pattern>/wel.do</url-pattern>
</servlet-mapping>
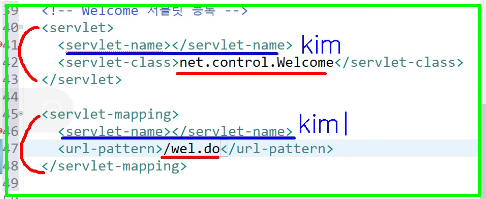
servlet-name은 두 개의 부분이 한 몸이라는 것을 연결해주기 위해 사용한다.
Welcome.java
package net.control;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/*
참조 https://docs.oracle.com/javaee/7/api/
● HttpServlet 클래스
- http 프로토콜 기반의 요청과 응답을 처리해주는 클래스
● 결과 확인
- http://localhost:9090/basic05_mvc/wel.do
● Welcome 서블릿 클래스
- web.xml (배치 관리자)에 등록해야 한다.
*/
public class Welcome extends HttpServlet{
//나의 클래스의 부모가 class : extends 상속(확장)
//나의 클래스의 부모가 interface : implements 상속(구현)
@Override //<- 재정의(리폼)
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//전송방식 method=get방식으로 요청하면 doGet() 함수가 자동으로 호출됨
//req : 모델1방식의 내부객체 request와 동일
//resp : 모델1방식의 내부객체 response와 동일
try {
//HTML 문서 구조로 응답
resp.setContentType("text/html; charset=UTF-8");
//단순 문자열 응답(AJAX)
//resp.setContentType("text/plain; charset=UTF-8");
//요청한 사용자에게 응답하기 위한 출력 객체
PrintWriter out = resp.getWriter();
out.print(" <!DOCTYPE html> ");
out.print(" <html> ");
out.print(" <head> ");
out.print(" <meta charset='UTF-8'> ");
out.print(" <title>환영합니다</title> ");
out.print(" </head> ");
out.print(" <body> ");
out.print(" <strong>대한민국</strong> ");
out.print(" <hr> ");
out.print(" <span style='color:red'>오필승코리아</span> ");
out.print(" <hr> ");
out.print(" <table border='1'> ");
out.print(" <tr> ");
out.print(" <th>이름</th> ");
out.print(" <td>무궁화</td> ");
out.print(" </tr> ");
out.print(" </table> ");
out.print(" </body> ");
out.print(" </html> ");
} catch (Exception e) {
System.out.println("요청실패 : " + e);
}//end
}//doGet() end
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//전송방식 method=post방식으로 요청하면 doPost() 함수가 자동으로 호출됨
}//doPost() end
}//class end
위에서 볼 수 있듯이 서블릿으로만 페이지를 만들면 HTML을 출력하는 것이 불편하다.
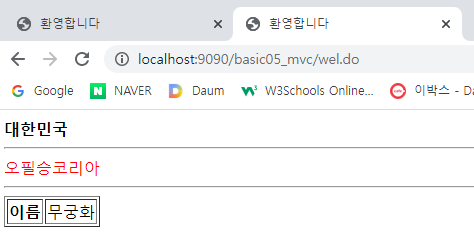
LifeCycle.java
package net.control;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/*
● HttpServlet 클래스의 계층도
class GenericServlet {}
class HttpServlet extends GenericServlet {}
class LifeCycle extends HttpServlet {}
● HttpServlet 생명주기-----------------------------
init() 서블릿이 호출되면 1번만 호출
-> service()
-> service()
-> service() 사용자가 요청할 때마다 계속 호출 (doGet()과 doPost()를 구분하는 역할)
-> service()
-> service()
destroy() 서버가 중지되면 1번만 호출
------------------------------------------------
● LifeCycle 서블릿을 /WEB-INF/web.xml에 등록해야 함
● 결과 확인 http://localhost:9090/basic05_mvc/life.do
*/
public class LifeCycle extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//method=get 방식으로 요청하면 service()함수가 호출함
super.doGet(req, resp);
System.out.println("doGet()호출...");
}//doGet() end
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//method=post 방식으로 요청하면 service()함수가 호출함
super.doPost(req, resp);
System.out.println("doPost()호출...");
}//doPost() end
@Override
protected void service(HttpServletRequest arg0, HttpServletResponse arg1) throws ServletException, IOException {
//URL을 통해서 요청이 들어오면, 전송방식이 method=get인지 method=post인지 판단해서
//get 방식이면 doGet(), post 방식이면 doPost()를 호출해 주는 함수
super.service(arg0, arg1);
System.out.println("service()호출...");
}//service() end
@Override
public void destroy() {
//서버가 중지되면 자동으로 1번만 호출
super.destroy();
System.out.println("destroy()호출...");
}//destroy() end
@Override
public void init() throws ServletException {
//서블릿이 최초로 호출될 때 1번만 호출
//초기 환경 설정할 때 사용
super.init();
System.out.println("init()호출...");
}//init() end
}//class end
web.xml
<!-- LifeCycle서블릿(HttpServlet 생명주기) 등록 -->
<servlet>
<servlet-name>lc</servlet-name>
<servlet-class>net.control.LifeCycle</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>lc</servlet-name>
<url-pattern>/life.do</url-pattern>
</servlet-mapping>
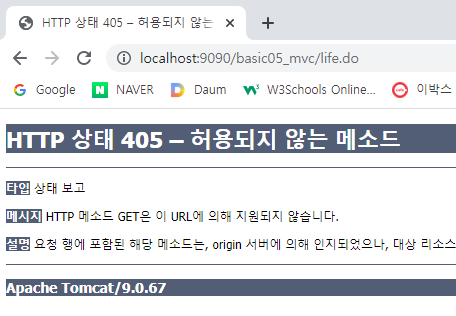
위 창을 새로고침 할 때마다
콘솔창에 doGet()호출... service()호출... 문구가 출력된다. 그리고 서버를 중지하면 destroy()호출... 문구가 출력된다.
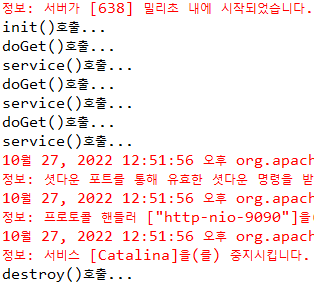
'웹개발 교육 > jsp' 카테고리의 다른 글
[65일] jsp (47) - MVC 패턴 (페이지 이동) (0) | 2022.10.28 |
---|---|
[64일] jsp (46) - MVC 패턴 (페이지 이동) (0) | 2022.10.27 |
[63일] jsp (44) - JSTL (0) | 2022.10.26 |
[63일] jsp (43) - EL (0) | 2022.10.26 |
[63일] jsp (42) - error (0) | 2022.10.26 |